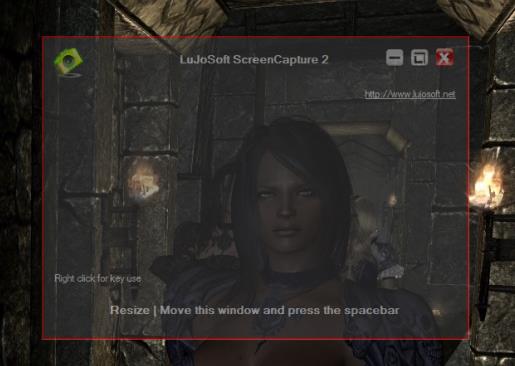
Introduction
Not much to say but to create this tool to capture any part and size of the screen you want, is very simple and can be accomplish in a few minutes.
Creating the application
1 - Create a new windows application project in Visual Studion, for this example I called it ScreeCapture, add a button and an OpenFileDialogue to the form.
2 - Add these using to the namespace
Code: Select all
using System;
using System.Drawing;
using System.Drawing.Imaging;
using System.Threading;
using System.Windows.Forms;
Code: Select all
private string strFilename;
Code: Select all
public string filename
{
get { return strFilename; }
set { strFilename = value; }
}
Now lets define the proprety for the OpenFileDialogue
Add this in the button event
Code: Select all
saveFileDialog1.Filter =
"JPG File (*.jpg)|*.jpg|BMP File (*.bmp)|*.bmp|PNG File (*.png)|*.png|GIF File(*.gif)|*.gif|Tiff File (*.tif)|*.tif";
saveFileDialog1.FilterIndex = 1;
saveFileDialog1.RestoreDirectory = true;
Code: Select all
if (saveFileDialog1.ShowDialog() != DialogResult.Cancel)
{
// Get the filename and store it
filename = saveFileDialog1.FileName;
}
else // If Cancel was selectedreturn
return;
Add
Code: Select all
// Creating new bitmap with the size of the form
Bitmap bmp = new Bitmap(this.Width, this.Height, PixelFormat.Format32bppArgb);
// Creating graphic object from the image
Graphics gr = Graphics.FromImage(bmp);
Code: Select all
gr.CopyFromScreen(this.Left, this.Top, 0, 0, this.Size, CopyPixelOperation.SourceCopy);
Code: Select all
switch (saveFileDialog1.FilterIndex)
{
case 1:
bmp.Save(filename, ImageFormat.Jpeg);
break;
case 2:
bmp.Save(filename, ImageFormat.Png);
break;
case 3:
bmp.Save(filename, ImageFormat.Bmp);
break;
case 4:
bmp.Save(filename, ImageFormat.Gif);
break;
case 5:
bmp.Save(filename, ImageFormat.Tiff);
break;
}
Hope you enjoy this article and you can download the full code in Visual Studio 2012 solution
[/align]